Let's build our first website
Chapter 2
Let's suppose that we want to build a webpage with a job posting. It should look something like this:
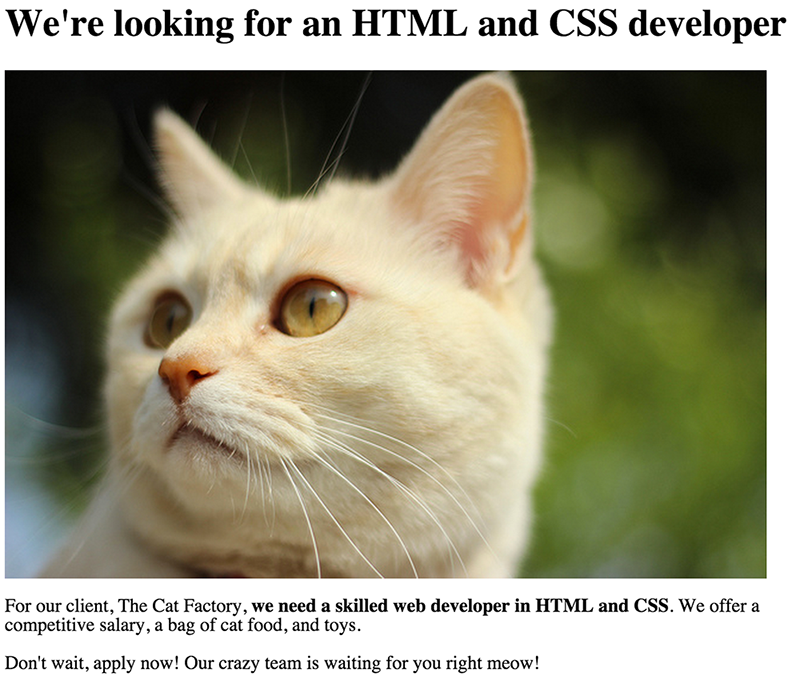
Before creating any kind of web page, it's a good idea to divide the content into smaller components by their importance. Let's now try to identify and highlight each element of this job posting, just as we did in the example from The Verge.
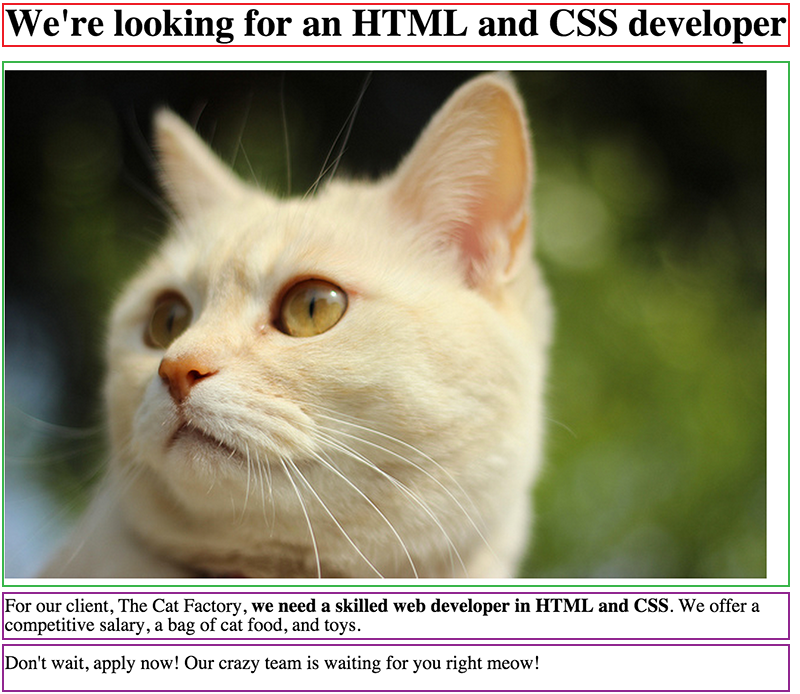
A brief analysis will help us to better understand which areas of the text should stand out in the job posting. Red indicates the headline text. Green indicates the accompanying image. And purple marks the two paragraphs (or "body") of the job posting.
Let's return for a moment to the analogy of word processors and text editing programs where web pages could be created in regular text documents. Perhaps Open, Notepad, or Word, would contain the text of the announcement, and then save as a text file. It should work right?
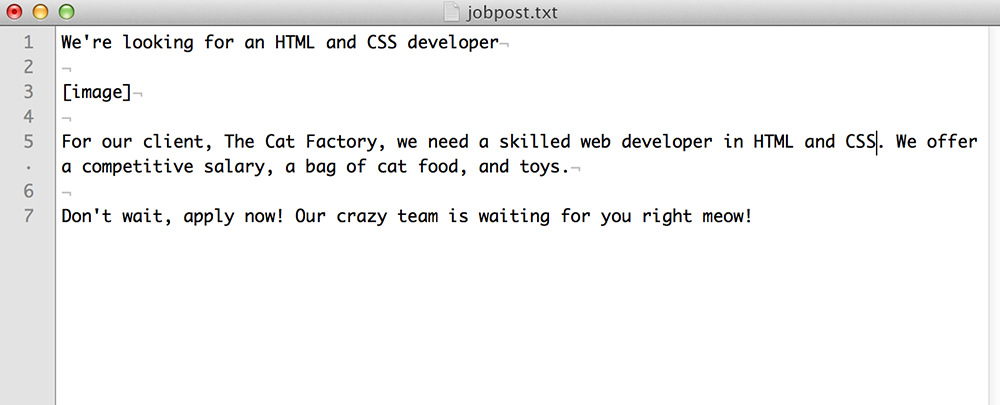
If you check this in a web browser, you get the following:
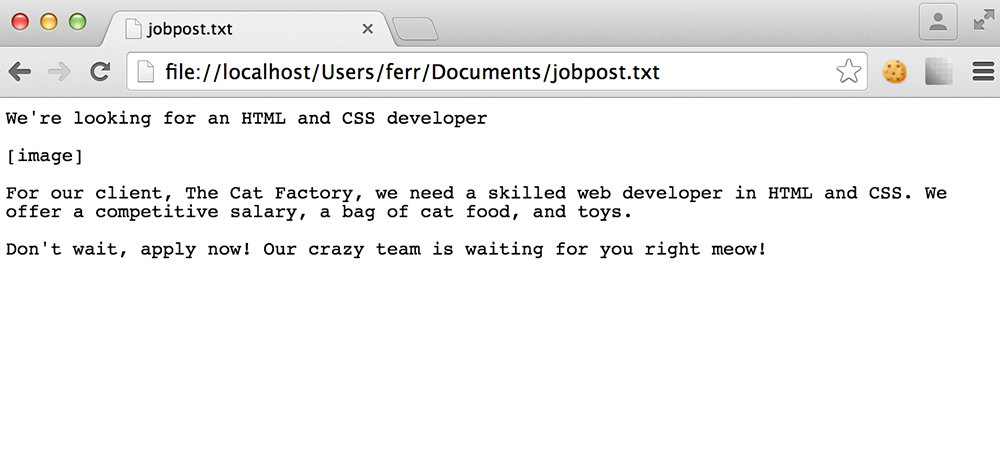
However, this doesn't look like what we designed, does it? It's just a mass of text and doesn't display a picture. What now? Maybe we should try to create this post in Word or Photoshop? We don't exactly want to do this. We've made an error here that we'll soon fix. The key problem is that we've created a website with only plain text.
A web browser cannot understand how to display a page properly with only plain text — it doesn't know which part of the text should be the title or which part should be a picture. In order to display the page properly, we need to define each element by the function of the text and pass this information to the browser. We've partly done it. Let's do a little definition work and use proper syntax so browser will also understand it:
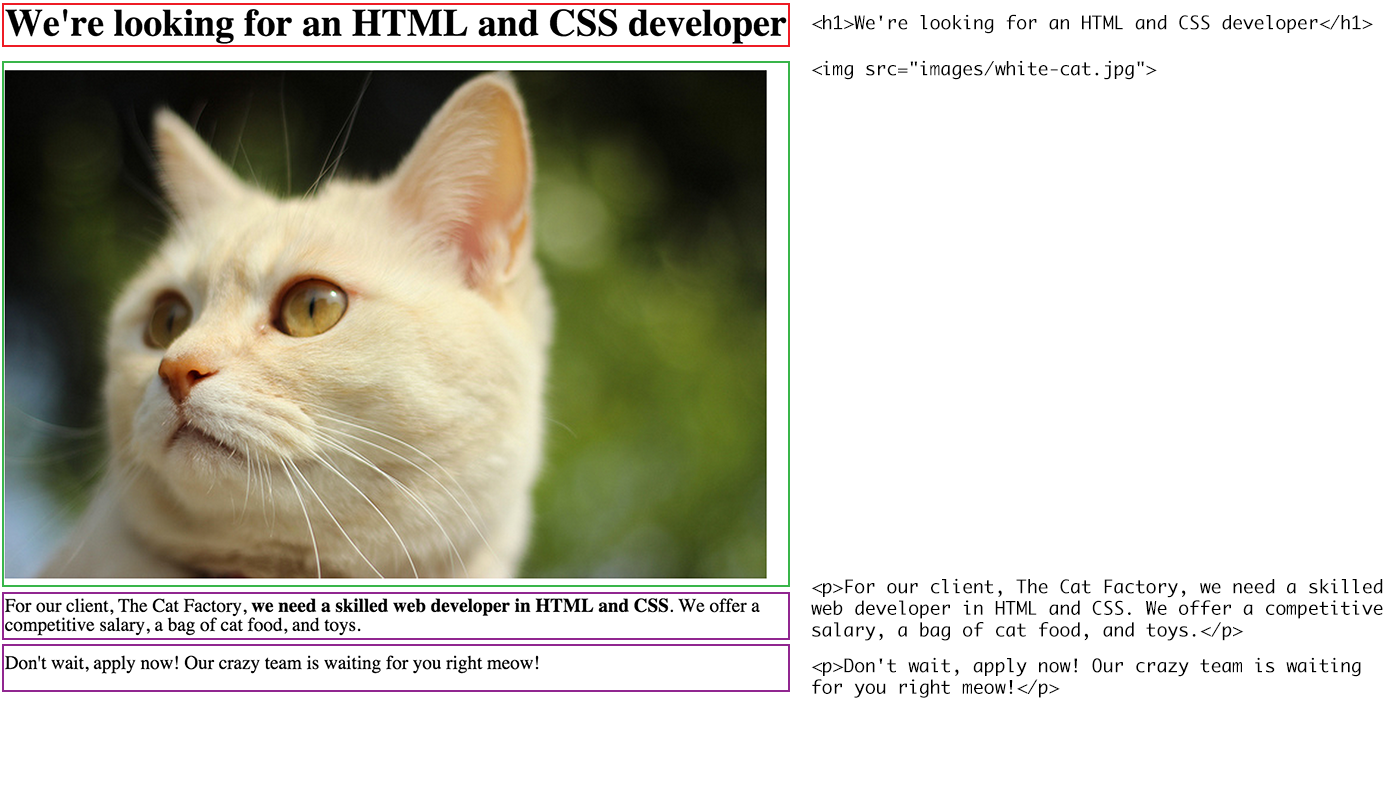
As you can see, there are special markings within the text. We'll get to these later. For now, it's best to simply try to copy them exactly as they are, from top to bottom, as if you were building with blocks, one by one.
You should get something like the following:
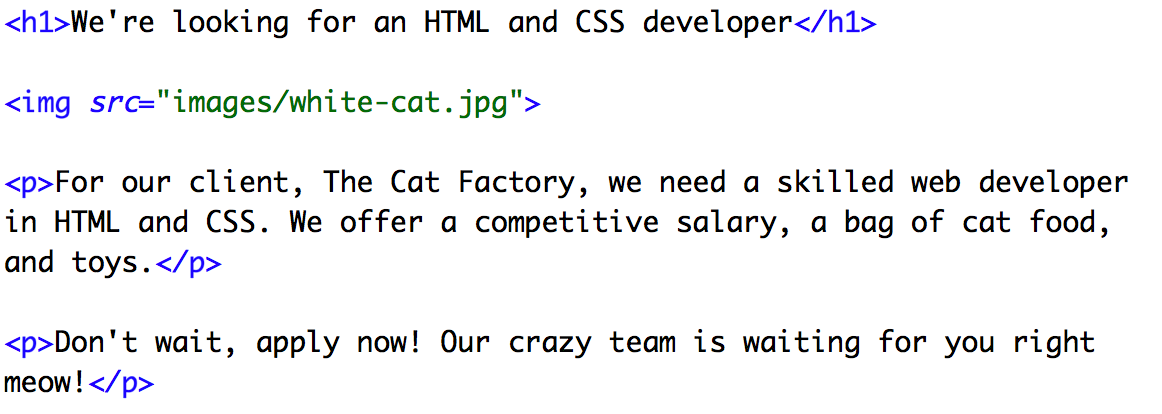
Next, save the HTML file. By the way: I recommend you Sublime Text Editor which is one of the best code editors out there.
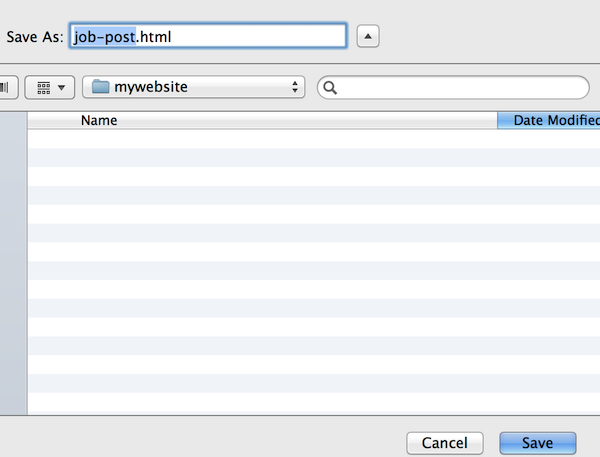
Now let's try the new file in a web browser.
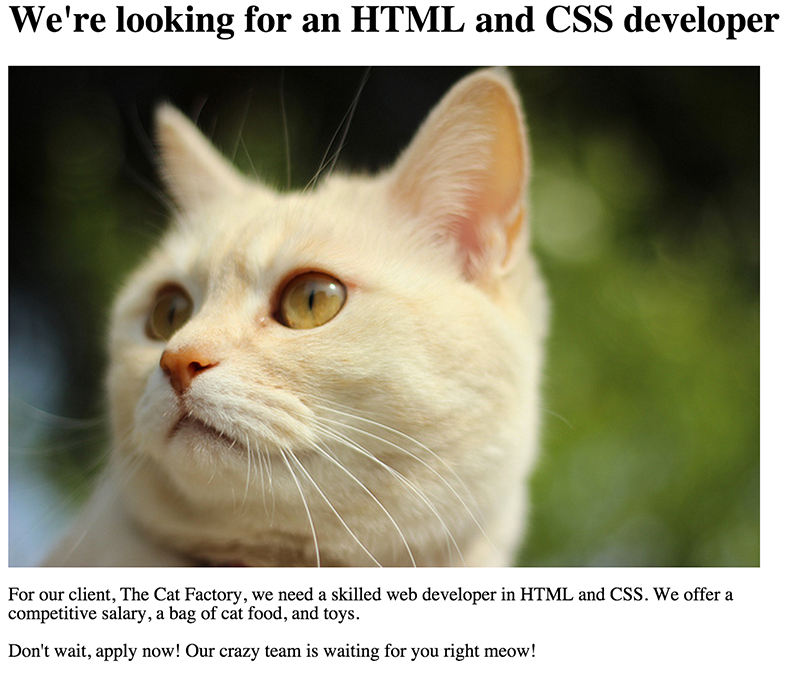
It looks a bit better. Each section of the text is now formatted differently, and the picture is also displayed. This is not the final version though. The lack of color, bold text and style doesn't quite match up yet. But before we work on that, let's keep our focus on the structure of the page.
First, let's compare the plain text document version of the posting, and the one presented in HTML:
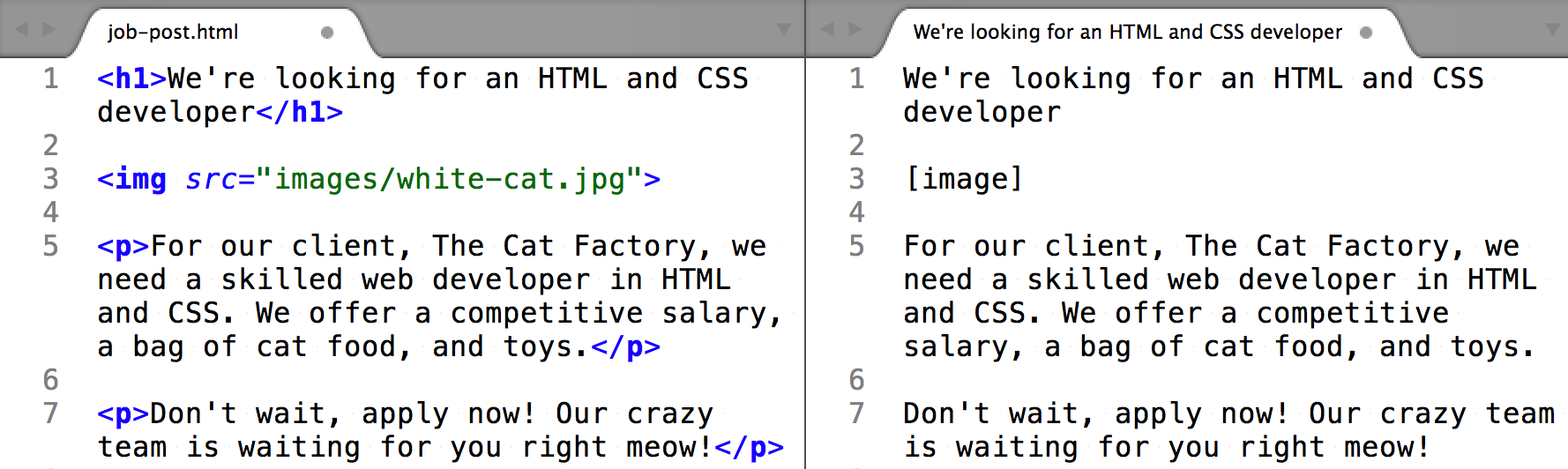
As a result of surrounding specific text fragments with special tags, we've created a completely different, which the browser understands. Way to go!
This is called HTML, or Hypertext Markup Language, and is the primary markup language for displaying information for a web browser. In simpler terms, it's a language that uses "tags" (like <this>) to mark text, so that you can describe the text to your browser. This also includes search engines and a few other programs which we'll cover later on. HTML tags are like grammar forms in verbal communication, without them, you cannot build a coherent website, much like you cannot form correct sentences or paragraphs without grammar.
In our case, we used only a few simple tags, and below is a list of the functions that each tag performs:
- <h1> - headline (of the first degree)
- <img> - picture element
- <p> - a paragraph of text
So, if you have a website with a few paragraphs of text, you surround each paragraph with the <p> tag. If the text is the main headline, then surround the text with <h1>, and so on and so forth.
All tag constructions are extremely simple and look like this:
<tagname>content</tagname>
You might have noticed that the second tag is different. This is because each tag must "close," as the saying goes in HTML jargon. The forward-slash appears in the closing tag to tell the browser that this marks the end of an element. For example, all paragraph elements end in </p>, while a Header 1 ends in </h1>.
There are also tags which do not need to be enclosed. For example image tags, <img>, which are used to insert pictures. In our example above, we used it as follows:
<img src="images/white-cat.jpg">
There are several more tags that do not need to to be "closed", which you will learn about later in this book.
One important realization to make about HTML tags is that, like dictionaries contain the vocabulary of a language, so too does HTML have a kind of dictionary that defines tags and describes when and where to use them. At the moment we have covered only a few, but there are many more. The international organization known as The World Wide Web Consortium (W3C) encourages best practices, standards, and compatibility across the web. You can view their definition of HTML elements here: http://www.w3.org/TR/html-markup/elements.html.
Also, its helpful to remember that, like natural languages, HTML evolves over time. Today, we use the fifth version of the HTML language, known as HTML5.
Returning to our example, it should look like the following in HTML code:
<h1>We're looking for an HTML and CSS developer</h1>
<img src="images/white-cat.jpg">
<p>For our client, The Cat Factory, we need a skilled web developer in HTML and CSS. We offer a competitive salary, a bag of cat food, and toys.</p>
<p>Don't wait, apply now! Our crazy team is waiting for you right
meow!</p>
Notice that it contains two paragraphs of text, marked within <p></p> tags:
<p>For our client, The Cat Factory, we need a skilled web developer in HTML and CSS. We offer a competitive salary, a bag of cat food, and toys.</p>
<p>Don't wait, apply now! Our crazy team is waiting for you right
meow!</p>
Suppose that we want the reader of our ad to pay particular attention to a certain part in a paragraph. Let's say that we wanted to mark the part of the text that says "we need a skilled web developer in HTML and CSS." How would an HTML tag let the browser know? The answer is the <strong> element, which will surround parts of text that should be represent strong importance for its contents.
<p>For our client, The Cat Factory, <strong>we need a skilled web developer
in HTML and CSS</strong>. We offer a competitive salary, a bag of cat food,
and toys.</p>
<p>Don't wait, apply now! Our crazy team is waiting for you right meow!</p>
Note that the <strong> tag surrounds the text, and also sits within the <p> tag. In programming terms, we would say that the <strong> tag is a "child" element within <p> because it nests within the parent. There are many tags that can nest within other tags, while many others cannot. Each tag has a specific list of possible HTML elements which it can contain (or be contained by), and you will need to check whether a certain tag is allowed or not. You can think of it like smaller blocks combining to make a bigger block piece (in this case the <strong> block is a component of <p>, which is in turn a block of the whole text).
Here is what it looks like on the web.

Take note that this web browser displayed the fragment in bold, but this is not always a rule. It's very common that <strong> makes text bold, but sometimes it will be ignored. The reason for using this HTML tag in this text is a good example of how nesting works, but visual effects (like bold) can be better achieved through a language called CSS, which we will learn more about in a moment.